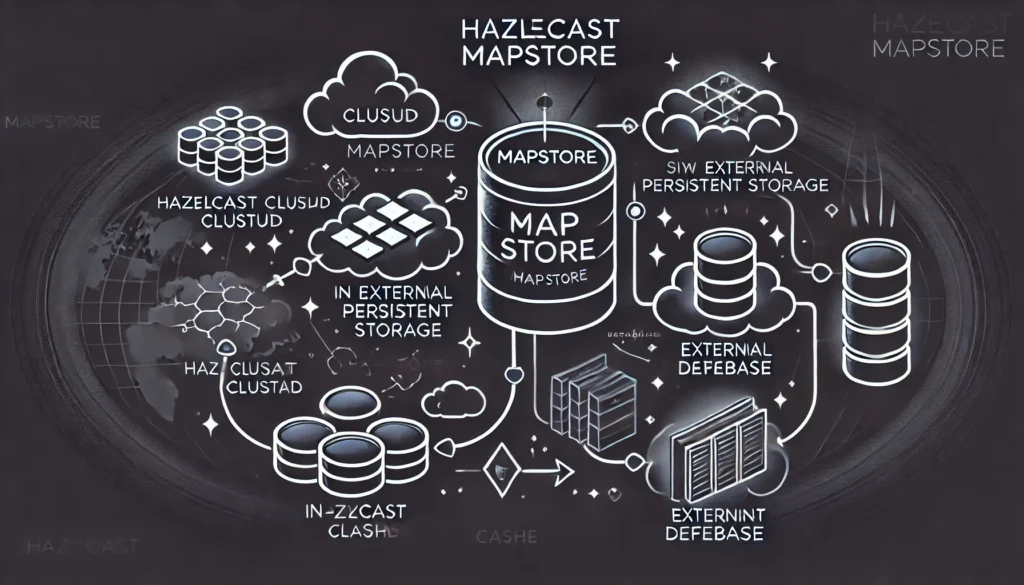
What is Hazelcast MapStore?
Hazelcast MapStore and MapLoader is an interface API to build a cache on Hazelcast. To build a cache, you can either use a pre-built component, which requires little or no coding. Or, you can use a custom option that allows you to write the interface yourself.
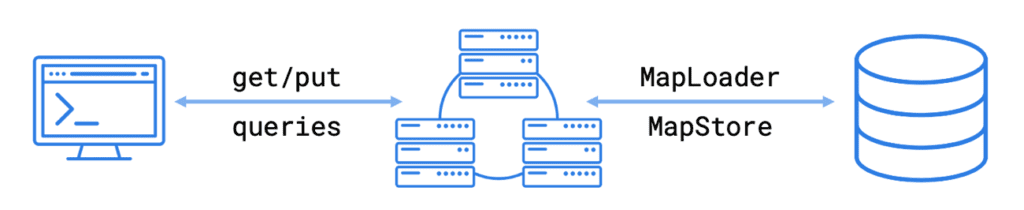
Hazelcast is in-memory data store, it can be backed persistence by any data store such as RDBMS, OODBMS, NOSQL, or simply a file-based data store. MapLoader is another API that re-load back data from database to in-memory map, check here for more detail about MapLoader.
IMap.put(key, value)
Normally stores the entry into JVM’s memory. If the MapStore implementation is provided then Hazelcast will also call the MapStore implementation to store the entry into a user-defined storage, such as RDBMS or some other external storage system. It is completely up to the user how the key-value will be stored or deleted.
IMap.remove(key)
Similar like java.util.Map operation MapStore has remove, the user can provide custom implement to delete.
Key Features of MapStore
- Persistence: Hazelcast MapStore writes the data into the database or file persistence storage, which ensures that the data will not be lost even if the distributed cluster restarts or shutdown.
- Lazy and Eager Loading: Hazelcast map configuration provides the feature of Lazy and Eager loading, it loads the data from storage at the time of map initialization or only if needed, as configured.
- Write-Through and Write-Behind: MapStore supports both write-through (synchronous) and write-behind (asynchronous) modes, it gives you flexibility in managing how data is synchronized with the backed persistent store.
How Does MapStore Work?
When we use the Hazelcast MapStore, Hazelcast intercepts operations on the IMap
API and connects with the persistent store as needed(refer to database connection pool and MapStore implementation). Here’s how it works:
- Loading Data: When a specific identity key is requested from Hazelcast
IMap
which is not yet loaded into distributed memory, MapStore API calls theload
method. This method retrieves the data from the database or filesystem persistent storage and loads it into the distributed map. - Storing Data: When new data is added to the
IMap
by using set() or put() API, MapStore’sstore
orstoreAll()
method gets invoked. Depending on the configuration, this happens synchronously or asynchronously. - Removing Data: When an entry is removed from the
IMap
, thedelete
method of MapStore is called to remove the entry from the persistent store.
Benefits of Using MapStore
- Data Consistency: By using MapStore, it maintains the consistency between Hazelcast distributed map and database persistent storage, also it ensures that no data is lost, as it is fail-safe.
- Scalability: MapStore enables your application to scale by offloading infrequently used data to a persistent store, keeping the in-memory footprint minimal.
- Flexibility: MapStore supports a variety of databases and storage systems, allowing you to choose the best fit for your application’s needs.
Hazelcast MapStore Mathods :
- void store(K key, V value) – Store the key value pair into Map.
- void storeAll(Map<K, V> map) – Collections of key and value, for multiple entries.
- void delete(K key) – Delete an specific key from Map.
- void deleteAll(Collection<K> keys) – Delete the collections of entries from MapStore.
Configuration of MapStore
Hazelcast MapStore can be configured by using XML, YML and Java, here you can use it for your custom mapstore implementation. com.demo.MyMapStore
is custom map store implementation class where you can @override above methods as you need.
Java
MapConfig mapConfig = new MapConfig("default"); 

MapStoreConfig mapStoreConfig = new MapStoreConfig(); 

mapStoreConfig.setClassName("com.demo.MyCustomMapStore"); 

mapConfig.setMapStoreConfig(mapStoreConfig);
XML
<hazelcast>

...

<map name="default">

<map-store enabled="true">

<class-name>com.demo.MyCustomMapStore</class-name>

</map-store>

</map>

...

</hazelcast>
YML
hazelcast:

map:

default:

map-store:

enabled: true

class-name: com.demo.MyCustomMapStore
However Hazelcast MapStore API calls method load() and store() operation to improve the throughput and the operations are offloaded.
This way partition threads are not blocked by them.
Each partition in a member is managed by a single thread, the write operations to a given partition are handled one at a time in first-in-first-out order. However, MapStore operations may take a long time to connect to the data store and complete, blocking the affected partitions. To avoid blocking partition threads, MapStore operations communicate with the data store asynchronously, you can implement ExecutorService.
<hazelcast> 

... 

<executor-service name="hz:map-store-offloadable"> 

<pool-size>16</pool-size> 

<queue-capacity>0</queue-capacity> 

</executor-service> 

... 

</hazelcast>
To block partition threads during MapStore API operations, set the offload
configuration to false
Configuring same MapStore in Multiple Maps
To initialize the MapStore with the given map name, configuration properties, and Hazelcast instance, the MapStore must implement the MapLoaderLifecycleSupport.
Config config = new Config();

MapConfig mapConfig = config.getMapConfig( "*" );

MapStoreConfig mapStoreConfig = mapConfig.getMapStoreConfig();

mapStoreConfig.setFactoryImplementation( new MapStoreFactory<Object, Object>() {

@Override

public MapLoader<Object, Object> newMapStore( String mapName, Properties properties ) {

return null;

}

}
);
Conclusion
Hazelcast MapStore implementation provides feature to store the data into RDBM or any other data store. You can perform MapStore operations and customize the implementation class. You can refer sample code snippet on github.
Pingback: Understanding of Hazelcast MapLoader – Java Tech ARC 3i
Pingback: What is Hazelcast QueueStore? - Java Tech ARC 3i
Pingback: How to integrate Hazelcast with Spring Boot? - Java Tech ARC 3i
Pingback: Hazelcast Hikari connection pooling
Pingback: Understanding Hazelcast Serialization and Deserialization : A Complete Guide JavaTechARC3i