Overview
Hazelcast is an in-memory data grid enabling developers to create highly scalable and distributed applications. The initial step to forming a cluster of nodes that can store and process data in-memory is to start a Hazelcast member (node) and a client. This article will discuss how to start Hazelcast members and clients and how to link them to establish a Hazelcast cluster.
To begin with starting Hazelcast Members and Client on Windows, you can opt for Docker, use the binary distribution, or run it through Java. Ensure that Hazelcast is correctly installed. You should be able to see the versions of both Hazelcast and the command-line client (CLI).
Pre-requisites:
- Download hazelcast, on demo I have used community addition.
- Java 8 or higher version, demo I have used Java17
- An IDE
Unzip the Hazelcast package go to /bin and Start Hazelcast Members and Client cluster, you can see the current system IP registered as member.
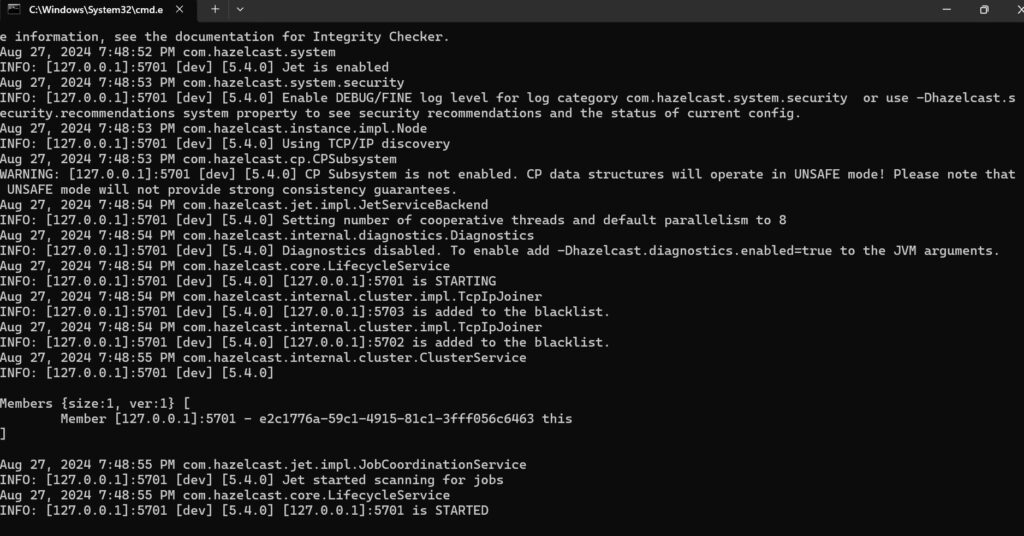
Starting Hazelcast Members
A Hazelcast member, also known as a node, is a key component of the Hazelcast cluster. Each member joins the cluster to share data and resources with other members. Here’s how to start a Hazelcast member:
Hazelcast client dependency
Add the below maven dependency in your project pom.xml or gradle file.
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>5.5.0</version> <!--Add the latest version-->
</dependency>
Gradle dependency:
implementation 'com.hazelcast:hazelcast:5.5.0'
Hazelcast connection config
To Start Hazelcast Members and Client If your client and cluster has configured on same system then the HazelcastInstance client would be autoconfigured. Also it can be integrated with Spring Boot
HazelcastInstance hz = HazelcastClient.newHazelcastClient();
But in real time scenario in eEnterprise world, clusters are installed on remote location, where multiple members may be connected with same cluster. So the client connection should be configured with member IP address.
ClientConfig config = new ClientConfig();
ClientNetworkConfig clientNetworkConfig = config.getNetworkConfig();
Properties properties = new Properties();
properties.put("hazelcast.instance", "dev");
properties.put("hazelcast.clustername", "hz-test");
properties.put("hazelcast.password", "");
clientNetworkConfig.setSSLConfig(new SSLConfig()
.setEnabled(false)
.setProperties(properties));
clientNetworkConfig.setAddresses(List.of("localhost:5701"));
config.setClusterName("dev");
config.setInstanceName("hz-test");
HazelcastInstance hazelcastInstance = HazelcastClient.newHazelcastClient(config);
In the above there are two configuraiton ClientConfig is main configuration of Hazelcast client and ClientNetworkConfig used for network member IP configurations.
Hazelcast Map
Hazelcast IMap<K, V>
is similar to java.util.Map
and it is concurrent, distributed, observable and queryable map.
This class is not a general-purpose ConcurrentMap
implementation! While this class implements the Map interface, it intentionally violates Map’s general contract, which mandates the use of the equals method when comparing objects. Instead of the equals method, this implementation compares the serialized byte version of the objects.
Moreover, stored values are handled as having a value type semantics, while standard Java implementations treat them as having a reference type semantics.
HazelcastInstance hazelcastInstance = HazelcastClient.newHazelcastClient(config);
IMap<String, String> testMap = hazelcastInstance.getMap("test-map");
System.out.println("Size before add : "+testMap.size());
testMap.put("Java", "JavaTechARC3i");
System.out.println("Size add add : "+testMap.size());
System.out.println("Map Data : "+testMap);
Complete client connection Code
public class HazelcastClientDemo {
public static void main(String[] args) {
ClientConfig config = new ClientConfig();
// Start the Hazelcast Client and connect to an already running Hazelcast Cluster on 127.0.0.1
//HazelcastInstance hz = HazelcastClient.newHazelcastClient();
ClientNetworkConfig clientNetworkConfig = config.getNetworkConfig();
Properties properties = new Properties();
properties.put("hazelcast.instance", "dev");
properties.put("hazelcast.clustername", "hz-test");
properties.put("hazelcast.password", "");
clientNetworkConfig.setSSLConfig(new SSLConfig()
.setEnabled(false)
.setProperties(properties));
clientNetworkConfig.setAddresses(List.of("localhost:5701"));
config.setClusterName("dev");
config.setInstanceName("hz-test");
HazelcastInstance hazelcastInstance = HazelcastClient.newHazelcastClient(config);
IMap<String, String> testMap = hazelcastInstance.getMap("test-map");
System.out.println("Size before add : "+testMap.size());
testMap.put("1", "JavaTechARC3i");
System.out.println("Size add add : "+testMap.size());
System.out.println("Map Data : "+testMap);
}
}
Output
Size before add : 0
Size add add : 1
Map Data : IMap{name='test-map'}
Conclusion
You can now start both Hazelcast members and clients, allowing you to create distributed applications using Hazelcast’s distributed data grid capabilities. With this setup, you can scale your cluster horizontally by adding more members and connecting clients to interact with the data. This is high level understanding of Start Hazelcast Members and Client about setting up the Start Hazelcast Members and Client cluster and connecting the clint.
The demo code available over the github
Happy Coding! 😊
Pingback: Understanding Hazelcast Serialization and Deserialization : A Complete Guide JavaTechARC3i