Overview
Protobuf Maven Plugin(maven-compiler-plugin
), allows user to working with Google Protocol Buffers aka Protobuff in maven base Java project, and integrate the Maven Plugin build tool is a good approach. To compile protocol buffer .proto
files and generate the required Java source code from compiler, user need to configure the Maven proto buffer build process to include a Maven Protobuf Plugin(maven-compiler-plugin) for Protocol Buffers.
The Protobuf Maven Plugin automates the compiler process to generate source code, allowing users to smooth compile .proto
files and package them into your Java application. The Maven Plugin setup perform automatically trigger the re-compilation during the project build and making easier to build the project as schema.
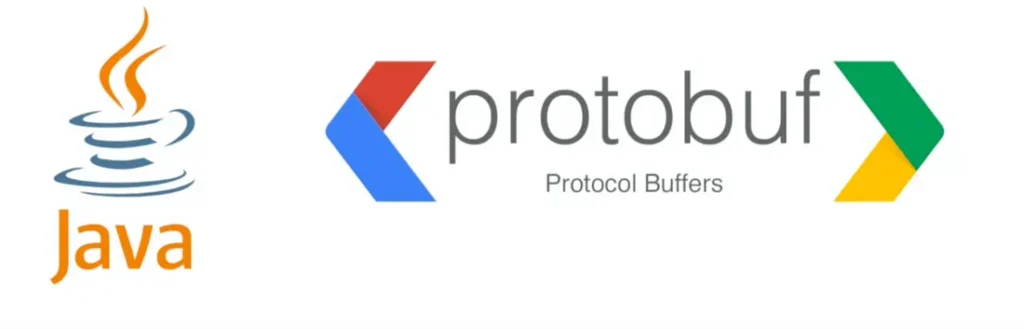
What is the Protobuf Maven Plugin?
The Protobuf Maven Plugin is a tool that compiles Protocol Buffers .proto
files into Java (or other supported languages) during the Maven build process. Instead of manually running the protoc
compiler for each change in your .proto
files, the plugin integrates with Maven’s lifecycle, automatically generating the required Java classes whenever you build your project.
Why Use the Protobuf Maven Plugin?
Protocol Buffers are commonly used for serializing structured data in Java applications, especially when building microservices, real-time applications, or distributed systems. Compiling .proto
files manually can be tedious and error-prone, especially in large projects. By using the Protobuf Maven Plugin, you can:
- Automate the compilation of
.proto
files into Java classes. - Ensure consistency across different development environments.
- Maintain schema updates seamlessly as part of your regular build process.
- Reduce build errors and manual overhead in managing Protobuf files.
Here’s how to set up Maven to handle Protocol Buffers using the protobuf-maven-plugin.
1. Adding Protobuf Maven Plugin
First, you need to configure the protobuf-maven-plugin
in your project’s pom.xml file. This plugin will automatically compile the .proto
files into Java source files during the Maven build process.
<build>
<plugins>
<!-- Maven Compiler Plugin -->
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<!-- Protocol Buffers Maven Plugin -->
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
<configuration>
<protocArtifact>com.google.protobuf:protoc:3.11.0:exe:${os.detected.classifier}</protocArtifact>
</configuration>
</plugin>
</plugins>
</build>
2. Dependency for Protocol Buffers
You’ll also need to include the Protobuf Java library as a dependency in your pom.xml. This library provides the runtime support for working with Protocol Buffers in Java.
<!-- https://mvnrepository.com/artifact/com.google.protobuf/protobuf-java -->
<dependencies>
<!-- Protocol Buffers Java runtime -->
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>4.28.1</version>
</dependency>
</dependencies>
3. Directory Structure for .proto
Files
By default, the protobuf-maven-plugin expects your .proto
files to be located in the src/main/proto
directory. This is where the plugin will look for any .proto
files that need to be compiled.
src/
main/
proto/
employee.proto
Make sure to create the necessary directory structure in your project and place your .proto
files in the src/main/proto
folder.
4. Compiling and Generating Code
Once your pom.xml is properly configured, you can use Maven’s standard build commands to compile the Protocol Buffers and generate Java code:
mvn clean compile
This command will trigger the protobuf-maven-plugin, which will compile the .proto
files into corresponding Java classes. These generated classes will be available in the target/generated-sources
directory, which will be included as part of the project’s source tree.
5. Using the Generated Code in Your Project
Once the .proto
files have been compiled, you can use the generated Java classes in your project. For example, if you have a Employee.proto
file that defines a message for a employee
, you can use the generated Employee class like this:
syntax = "proto3";
message Employee {
string id = 1;
int32 name = 2;
string email = 3;
}
Employee employee = Employee.newBuilder()
.setId(1234)
.setName("Java Tech ARC 3i")
.setEmail("java@javatecharc.com")
.build();
byte[] serializedData = employee.toByteArray();
Employee deserializedEmployee = Employee.parseFrom(serializedData)
Advanced Configuration
The protobuf-maven-plugin supports advanced configurations, such as customizing the directories for .proto
files or generating code in languages other than Java. You can tweak the plugin’s behavior to suit your specific project needs.
For instance, to customize the output directory of the generated sources, you can modify the configuration:
<configuration>
<outputDirectory>${project.build.directory}/generated-sources/protobuf</outputDirectory>
</configuration>
You can also generate code for languages like Python, C++, and Go by adjusting the goals and configurations in your pom.xml
file.
Benefits of Using the Protobuf Maven Plugin
1. Automated Workflow
The Protobuf Maven Plugin integrates directly with Maven’s build lifecycle, eliminating the need for manual compilation of .proto
files. This automation ensures that every time you build your project, any changes to .proto
files are immediately reflected in the generated code.
2. Increased Efficiency
By generating Java classes from .proto
files automatically, the plugin speeds up the development process and reduces the likelihood of errors. Developers can focus on writing business logic without worrying about keeping the generated classes in sync with the .proto
definitions.
3. Multi-Language Support
While this blog focuses on Java, the Protobuf Maven Plugin supports multiple languages. This flexibility allows teams using different programming languages to collaborate seamlessly, as the same .proto
schema can be compiled into different languages.
4. Schema Evolution
One of the key strengths of Protocol Buffers is their support for backward and forward compatibility. The Protobuf Maven Plugin helps you manage schema changes efficiently by ensuring that generated code is up-to-date with the latest .proto
definitions, making it easier to evolve your system over time.
Conclusion
The Protobuf Maven Plugin is an essential tool for any Java project that utilizes Google Protocol Buffers. By automating the process of compiling .proto
files and generating Java classes, it streamlines development and ensures that your project stays synchronized with your Protobuf schema. Whether you’re building a microservice architecture, working on real-time systems, or developing a distributed application, the Protobuf Maven Plugin can help you manage data serialization effectively and efficiently.
To get started, simply integrate the plugin into your pom.xml
and enjoy the benefits of automatic Protobuf code generation in your Maven-based projects. To know more visit Overview of Google Protocol Buffers.
Table of Contents
- Working with Google Protocol Buffers com.google.protobuf.Timestamp and java.time.LocalDateTime
- Understanding Google Protobuf Timestamp
- Data Types in Protocol Buffers(Protobuf): A Complete Guide
- Protobuf Maven Plugin for Protocol Buffers
- Google Protocol Buffers Explained: How They Make Your Applications Run Smoother