Overview
Java is a popular programming language among the developers, it is a starting point for beginners in development who are their career in programming.
Exception Handling is an important concept in Java programming that provides features to protect your code to break from runtime errors. If any error occurs in the program, then we can use the Java exception handling mechanism to execute the program smoothly.
In this article, we will explore Java Exception handling concepts in Java with examples and how to use them effectively in Java projects.
Also explore:
- How to Install Java: A Complete Step-by-Step Guide
- How to Set Java Classpath?
- Java Hello World! Tutorial: The Beginner’s Entry to Coding
What is Exception Handling concepts in Java?
Java Exception handling concept is an unexpected event which occurs at runtime during the program execution. There are many typical cause during runtime which disrupts normal execution flow of Java program.
For example:
- File not found
- Invalid input
- Division by zero
- Code error
- Exceeding memory limits
- Interruption on network connectivity
Note: Exceptions are different from errors. Errors cannot be handled, and the program gets stopped. But exceptions can be handled at runtime.
Hierarchy of Exceptions Classes in Java
Java exception handling concepts in Java, exceptions are under the hierarchy Throwable. Throwable is the superclass of all exceptions and errors. The exceptions are classified into two categories checked and unchecked exceptions. The exception hierarchy included the following classes:
- RuntimeException
- ArithmeticException
- ArrayStoreException
- ClassCastException
- IllegalArgumentException
- CloneNotSupportedException
- InterruptedException
- ReflectiveOperationException
- ClassNotFoundException
- IllegalAccessException
- InstantiationException
- NoSuchFieldException
- NoSuchMethodException
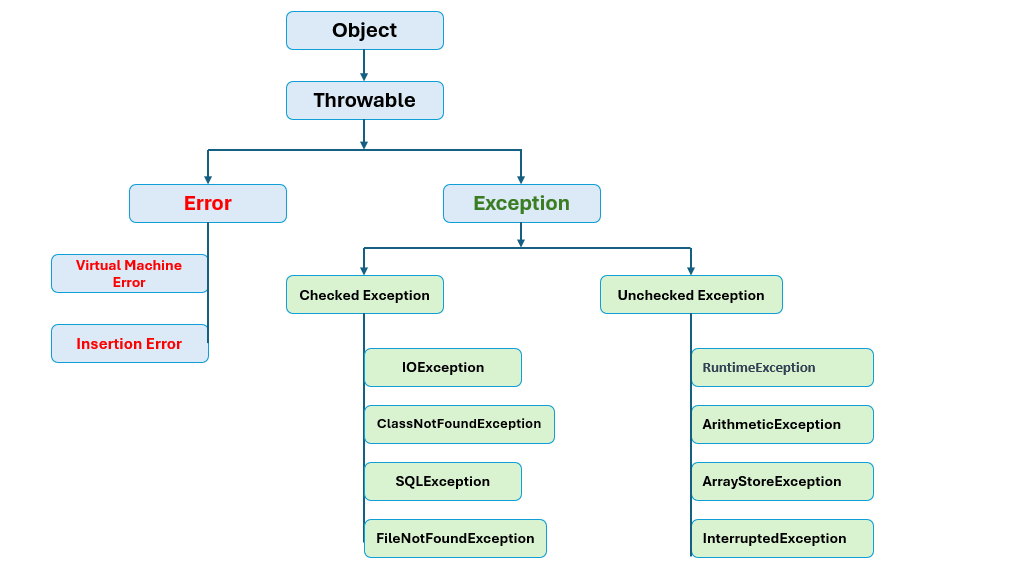
Java Exception Types
In Java exception handling concepts, there are two primary types of Exceptions:
1. Checked Exception
Checked exceptions are specific blockers, on the program compilation and are checked at compile-time. The compiler verifies the code that must be handled either using catch or adding throws in the method signature.
Example:
package com.javatecharc.demo;
import java.io.*;
public class CheckedExceptionExample {
public static void main(String[] args) {
try {
FileReader reader = new FileReader("file.txt");
} catch (FileNotFoundException e) {
System.out.println("File not found: " + e.getMessage());
}
}
}
- IOException: It is thrown, when input/output operations fails.
- FileNotFoundException: This is a subset of
IOException
, that is thrown while reading/writing a file and if the specified file is not available on given file system. - SQLException: Occurs if any failure in database operation
2. Unchecked Exception
Unchecked executions also known as a runtime exception, are not checked at compile time. It cannot be verified by the compiler and cannot be declared in method signature. This kind of exception usually occurs due to programming, logic, and incorrect assumptions.
Code scenario example:
public class UncheckedExceptionExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
System.out.println(numbers[5]); // ArrayIndexOutOfBoundsException
}
}
- NullPointerException: Thrown when object or reference are null and trying to get value from it.
- ArrayIndexOutOfBoundsException: Thrown when you are trying to access an invalid element of an array.
- ArithmeticException: Thrown when arithmetic operation fails, for example divided by value zero.
Exception Handling Keywords in Java
Java has given some keywords which, which can be used to handle the exceptions:
1. try
The try
block can used on the code where exceptions can occur or are thrown. But try
block can not be written alone, it must be written either with catch or finally block.
2. catch
The catch
block used to handle the exception that has occurred from try
block. It must be written with the try
block and optional with finally
block
Example:
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero.");
}
3. finally
The finally
block is also known as the resource handler. For example, if you want to close the connection or I/O stream, after every program execution upon program success or failure, then the code can be written into a finally
block. In finally
block write the necessary code that must be executed, either case of successful execution or failing of a program.
Example:
Socket s = new Socket();
try {
s.getInputStream();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
s.close();
} catch (IOException e) {
e.printStackTrace();
}
}
4. throw
The throw
keyword is used to manually throw the exceptions, which can be handled by try-catch or propagating to the next layer of application.
Example:
public void checkNumber(int num) {
if (num < 0) {
throw new IllegalArgumentException("Number cannot be negative.");
}
}
In the above code snippet, if num<0
then throw the IllegalArgumentException
5. throws
The throws
keyword is the prevention of exception, it must be declared as part of the method signature. It specifies there may be exceptions that occur at runtime, so it gives the warning before the usage of a specific method.
Example:
public void readFile(String fileName) throws IOException {
//code that may throw an IOException
}
Create Custom Exceptions
Java provides many in-built Exception classes, it also allows to creation of custom exceptions by extending the Exception
or RuntimeException
class.
Exception:
class JavaTechARCCustomException extends Exception {
public JavaTechARCCustomException(String message) {
super(message);
}
}
public class MyCustomExceptionExample {
public static void main(String[] args) {
try {
throw new JavaTechARCCustomException("This is a demo custom exception.");
} catch (JavaTechARCCustomException e) {
System.out.println(e.getMessage());
}
}
}
JavaTechARCCustomException
is custom exception class, extends Exception class.- It is mandatory to extend the Exception or
RuntimeException
class - Create at least one constructor and call
super()
inside the constructor.
Best Practices for Java Exception Handling
The following are the best practices for clean and efficient coding:
1. Catch Specific Exceptions
Catch specific exceptions whichever is possible to occur in your program and provide the specific error message, which will help you to debug your exceptions in case of any issue.
2. Use Finally for Cleanup
Always use finally
block for resource cleanup. For example – close the database connection, I/O stream closing, etc.
3. Avoid Swallowing Exceptions
Try re-throwing the exception in another part of the program; it should not stop on the backed layer.
4. Log Exceptions
Log the exception with valid exception messages, it should provide the proper information to the end user about occurred exceptions.
5. Use Custom Exceptions Wisely
Use the custom exception as your application’s specific. It should have information about exceptions and layers, which would be helpful and understandable during the debug process.
Conclusion
In Java exception handling concepts are a strong mechanism that makes your application more reliable and user-friendly. By using checked exceptions, and custom exception concepts, you can build your application more robust, and easy to debug in case of any issue. Good exception handling not only handles the exception, it also improves the user experience.
Happy Coding! 😊