Explore our Java Beginner Tutorials to master the basics of Java programming. Ideal for newcomers, these resources will help you build a strong foundation in Java programming effortlessly!
What is Java?
Java is the most popular, high-level programming language which is platform-independent, which means once you write the program and run it on any platform like Windows, macOS, or Linux. Java was developed by Sun Microsystem (currently part of Oracle) in 1995.
The core principle of Java is well known as “Write Once, Run Anywhere”, which means once you write a Java program you can run it anywhere without any modification.
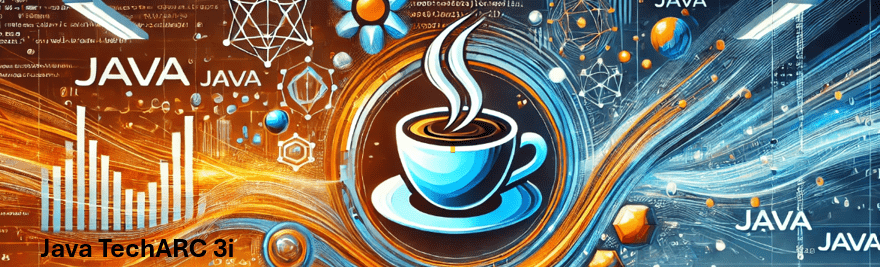
Key Features of Java:
- Object-Oriented: Java is an Object-Oriented Programming (OOP) language, where code can be organized in form of object and classes. This approach give the codes reusability, scalability and maintainability
- Platform Independent: Java compiles the code into bytecode that can run on any platform through Java Virtual Machine (JVM), as it gives the feature of platform independent.
- Simple: Java architecture design inspired by C++, but complex feature from C++ like pointers and multiple inheritances has been removed from Java, which is made it very easy to learn.
- Secure: Java has security features which is bytecode verification and runtime security checks, which give us secure environment to run the program.
- Robust (Strong): Java has a robust error handling and exception handling features, also it has automatic garbage collection which clean unwanted memory usages.
- Multithreaded: Java support multithreading, which give us the capability to efficiently execute the multiple tasks at a time. It is very useful for application where we require the concurrency features.
- Community and Support: Java has huge community, where developer can share their knowledge, and they get wide selection of libraries and tools.
Usage of Java:
- Web Development: Java can used for develop the web applications like JSP (Java Server Pages) and Servlets.
- Mobile Applications: Java can be used on mobile app development, where Android apps primarily written in Java.
- Enterprise Applications: Java used on large-scale enterprise-level applications like banking systems, business applications, etc.
- Scientific Applications: Java can be used complex scientific applications, mathematical computations, and data analysis apps.
- Embedded Systems: Java can be used embedded systems like smart devices, IoT applications, etc.
- Open-Source Frameworks: Java used on many open-source framework like Spring, Hibernate, EJB, Hazelcast etc.
Java’s Components:
- JDK (Java Development Kit): JDK provide development kit for programming language which help us to develop, compile, debug, run the program and many other features for development.
- JRE (Java Runtime Environment): JRE is run time environment, which provide the libraries to execute the program at runtime.
- JVM (Java Virtual Machine): JVM is engine which help us to run the bytecode. JVM is different for each platform, that convert the bytecode to machine language to run the code on specific platform.
Java Syntax Example:
To write the java code we need to follow a basic structure. Here is the example:
public class MyFirstProgram {
public static void main(String[] args) {
System.out.println("Welcome to Java Programming!");
}
}
- Main Method: The
main
method is the entry point of any Java application. It is the start point of program execution.
- Variables and Data Types: Java supports various data types, such as
int
,float
,char
,boolean
, andString
. Variables must be declared with a type before use.
Welcome to Java Programming!
- Java Tutorials
Java Versions
- Java 8
- Java 11
- Java 17
- Java 21
Java Basics
- Install JDK
- Set Java Classpath
- Hello World Program
- JDK, JRE, JVM and Differences
- Java Object-Oriented Programming (OOP) Concept
- Java Naming Rules
- Java Exception Handling
Java Streams
Java Stream API provides a feature to process and retrieve data from collections using terminal and non-terminal operations.
- Stream API
- Functional Interface
- Default and Static Methods
- Lambda Expressions