Overview
Monitoring a distributed system like Hazelcast is crucial to maintaining high availability and performance. While Hazelcast provides robust tools like Management Center, one of the most flexible methods for monitoring is through the REST API. This approach offers real-time, customizable insights into the health and performance of your Hazelcast cluster. In this blog, we’ll explore how to effectively Hazelcast REST API Monitoring API, covering essential endpoints, integration strategies, and best practices.
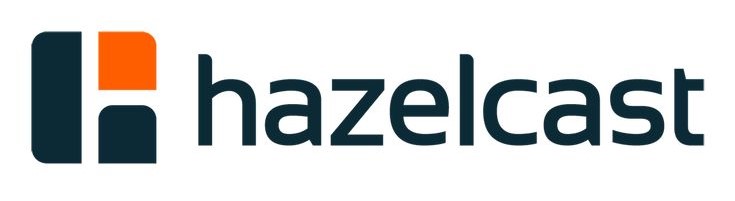
Why Use the REST API for Hazelcast Monitoring?
Hazelcast REST API Monitoring offers several benefits that make it a compelling option for monitoring:
- Flexibility: The API can be easily integrated into existing monitoring frameworks, custom dashboards, or alert systems.
- Real-Time Insights: Provides up-to-the-second data about nodes, memory usage, partitions, and more.
- Customizability: You can pull exactly the metrics you need, reducing overhead and complexity compared to broader tools like Management Center.
- No Additional Overhead: No need to install third-party tools—Hazelcast’s REST API can be queried from any system capable of making HTTP requests.
How to Enable the Hazelcast REST API
By default, Hazelcast REST API Monitoring is disabled for security reasons. To enable it, you must configure it in the hazelcast.xml
file.
Here’s how to enable REST API access:
<hazelcast>
<network>
<rest-api enabled="true">
<endpoint-groups>
<health-check enabled="true"/>
<cluster-read enabled="true"/>
<cluster-write enabled="false"/>
</endpoint-groups>
</rest-api>
</network>
</hazelcast>
In this configuration:
- The
health-check
andcluster-read
endpoints are enabled to allow read-only access, ensuring you can monitor the system without exposing write functionality. cluster-write
is disabled to protect the system from unintended or malicious changes.
Java Environment Setup:
You need a Java development environment (JDK 8 or higher) and a build tool like Maven or Gradle for dependency management.
Using Java’s HttpURLConnection
for REST API Requests
Java’s built-in HttpURLConnection
class is an easy and lightweight way to interact with REST APIs. Here’s how you can use it to query Hazelcast’s REST API.
Key REST API Endpoints for Monitoring
Once the Hazelcast REST API Monitoring is enabled, you can start querying Hazelcast’s endpoints to gather critical metrics about your cluster. Below are the most important REST API endpoints to monitor.
1. Health Check Endpoint
The health check endpoint is a simple but crucial endpoint that provides a binary view of the cluster’s health.
- URL:
GET /hazelcast/health
- Possible Responses:
200 OK
: The cluster is healthy.503 Service Unavailable
: The cluster is unhealthy.
You can integrate this endpoint into monitoring systems like Prometheus or Nagios to trigger alerts when the cluster health status is 503
, indicating a failure or degradation in performance.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HazelcastRestMonitorDemo {
private static final String HAZELCAST_URL = "http://localhost:5701/hazelcast/health";
public static void main(String[] args) {
try {
URL url = new URL(HAZELCAST_URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == 200) {
System.out.println("Cluster is healthy.");
} else {
System.out.println("Cluster is unhealthy. Status code: " + responseCode);
}
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println("Response: " + response.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
2. Cluster Information Endpoint
This endpoint provides detailed information about the overall state of the cluster, including the number of active nodes and partitions.
- URL:
GET /hazelcast/rest/cluster
The response includes:
- Cluster state (active, frozen, passive)
- Number of nodes in the cluster
- Version of Hazelcast
This information is critical for ensuring that your cluster is running as expected and that all nodes are correctly participating.
3. Node Information Endpoint
The node information endpoint provides detailed statistics about the individual nodes within the Hazelcast cluster, including CPU usage, memory consumption, and the status of each node.
- URL:
GET /hazelcast/rest/nodes
This is particularly useful for monitoring the performance of individual nodes and detecting imbalances or failures.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HazelcastNodeMonitorDemo {
private static final String NODES_URL = "http://localhost:5701/hazelcast/rest/nodes";
public static void main(String[] args) {
try {
URL url = new URL(NODES_URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == 200) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Display node details
System.out.println("Node Info: " + response.toString());
} else {
System.out.println("Failed to retrieve node information. Status code: " + responseCode);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
4. Partition Information Endpoint
Partitions are essential to Hazelcast’s distributed architecture. This endpoint gives you detailed information about partition ownership and distribution across the cluster.
- URL:
GET /hazelcast/rest/partition
The response contains:
- Number of partitions
- Which nodes own which partitions
- Partition state (active, migrating, etc.)
Monitoring partition distribution ensures that data is evenly distributed across nodes and that no node is overloaded or underutilized.
5. Memory Usage Endpoint
Memory is a critical resource in any Hazelcast cluster, as it operates primarily in-memory. This endpoint provides statistics about heap and off-heap memory usage.
- URL:
GET /hazelcast/rest/memory
Metrics provided include:
- Total memory available
- Memory in use
- Garbage collection statistics
This endpoint helps prevent memory overconsumption and ensures that your nodes are not at risk of running out of memory.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HazelcastMemoryMonitorDemo {
private static final String MEMORY_URL = "http://localhost:5701/hazelcast/rest/memory";
public static void main(String[] args) {
try {
URL url = new URL(MEMORY_URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == 200) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Parse or log the memory metrics as needed
System.out.println("Memory Usage: " + response.toString());
} else {
System.out.println("Failed to get memory usage. Status code: " + responseCode);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
6. Map and Queue Statistics Endpoint
Hazelcast’s distributed maps and queues are some of its most commonly used data structures. Monitoring them ensures that your application runs efficiently.
- URL (for a map):
GET /hazelcast/rest/maps/{mapName}
- URL (for a queue):
GET /hazelcast/rest/queues/{queueName}
The response includes:
- Number of entries in the map or queue
- Cache hits and misses
- Read and write operation counts
Monitoring this data helps ensure optimal performance and identify hot spots or potential bottlenecks in your application.
Maven Dependency for Jackson
Add the following dependency to your pom.xml
if you’re using Maven:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version>
</dependency>
Example of Parsing JSON with Jackson
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HazelcastJsonMonitorDemo {
private static final String MEMORY_URL = "http://localhost:5701/hazelcast/rest/memory";
public static void main(String[] args) {
try {
URL url = new URL(MEMORY_URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == 200) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Parse the JSON response
ObjectMapper mapper = new ObjectMapper();
JsonNode rootNode = mapper.readTree(response.toString());
// Example: Extract total and used memory
long totalMemory = rootNode.path("total").asLong();
long usedMemory = rootNode.path("used").asLong();
System.out.println("Total Memory: " + totalMemory);
System.out.println("Used Memory: " + usedMemory);
} else {
System.out.println("Failed to get memory usage. Status code: " + responseCode);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Best Practices for Hazelcast REST API Monitoring
To ensure effective monitoring through the REST API, follow these best practices:
- Set Up Alerts for Critical Metrics
Establish thresholds for essential metrics like memory usage, CPU load, and cluster state. Alerts should trigger well before these limits are breached, allowing for proactive resolution of issues. - Secure the REST API
Ensure that the REST API is secured using HTTPS, and restrict access to the API by implementing authentication and role-based access controls (RBAC) where necessary. - Monitor Regularly, But Avoid Overloading the API
Set reasonable intervals for polling the API. Overly frequent requests can put unnecessary load on your Hazelcast cluster and network. A typical interval is 10–30 seconds, depending on your monitoring needs. - Log Historical Data
While Hazelcast’s REST API provides real-time monitoring, logging historical data is also important. This allows you to detect trends, identify slowdowns over time, and make more informed decisions about capacity planning. - Combine Hazelcast REST API Monitoring with Other Monitoring Tools
Use Hazelcast’s REST API alongside other monitoring solutions like Management Center, JMX, or third-party monitoring platforms. This ensures that you get a comprehensive view of your cluster’s health and performance.
Conclusion
By writing custom monitoring scripts in Java, you can easily integrate Hazelcast REST API Monitoring into your existing infrastructure. Whether it’s checking the cluster health, monitoring memory usage, or gathering node-specific information, these scripts provide real-time insights to keep your system running smoothly. With Java’s HttpURLConnection
and libraries like Jackson, you can fetch, parse, and act on this data as needed. Also explore about Hazelcast JMX Monitoring The exmaple code available here in github.