Overview
Hazelcast is a powerful in-memory data grid that helps manage data across distributed systems. One of its key features is listeners, which allow you to handle events efficiently in real-time. Whether you’re adding entries to a map, publishing messages to a topic, or updating a distributed queue, Hazelcast listeners ensure that these events trigger immediate actions across the system.
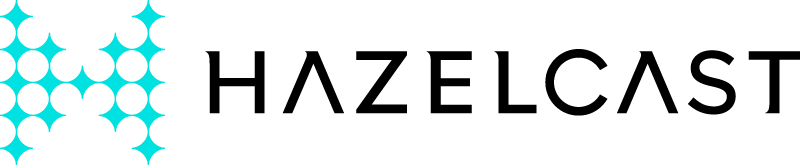
In this blog post, we’ll explore the types of Hazelcast listeners, how to configure them, and how they contribute to efficient event handling in distributed environments.
What are Hazelcast Listeners?
In a distributed system, events such as data updates or message publications happen across multiple nodes. Hazelcast listeners provide a way to monitor these events and respond in real-time. They are essentially event handlers that trigger specific actions when an event occurs, such as adding an entry to a distributed map or publishing a message to a topic.
These listeners play a key role in maintaining consistency and responsiveness within a Hazelcast cluster. By immediately reacting to changes, they help ensure that all nodes stay in sync and that necessary updates are applied uniformly across the system.
Types of Hazelcast Listeners
There are several types of listeners in Hazelcast, each designed for specific events and use cases:
- MapListener MapListener allows monitoring of changes in distributed maps. It can track events such as updates, removals, and additions, offering more flexibility compared to EntryListener.
- EntryListener The EntryListener is used to monitor changes in maps. It tracks events such as entry creation, removal, update, or expiration.
- ItemListener The ItemListener is designed for collections like queues, sets, and lists. It notifies applications when an item is added or removed from these structures.
- MigrationListener This listener is essential in distributed systems. It monitors partition migration between Hazelcast nodes, ensuring data consistency and high availability during node rebalancing.
- LifecycleListener The LifecycleListener tracks the lifecycle events of the Hazelcast instance. It is useful for monitoring critical events such as node shutdown, start, and restart.
Configuring Hazelcast Listeners
Setting up a listener in Hazelcast is simple and can be done either programmatically or through XML configuration. Let’s look at how to configure an EntryListener programmatically:
Create DemoEntryListener
listener and implement EntryAddedListener<K, V>
import com.hazelcast.core.EntryEvent;
import com.hazelcast.map.listener.EntryAddedListener;
public class DemoEntryListener implements EntryAddedListener<Integer, String> {
@Override
public void entryAdded(EntryEvent entryEvent) {
System.out.println("Do something for entry added event... ");
}
}
In this example, an EntryAddedListener
is added to a map to monitor when new entries are added. The entryAdded
method triggers each time an entry is added, allowing you to take immediate action based on the event.
import com.hazelcast.client.HazelcastClient;
import com.hazelcast.client.config.ClientConfig;
import com.hazelcast.client.config.ClientNetworkConfig;
import com.hazelcast.collection.IQueue;
import com.hazelcast.config.SSLConfig;
import com.hazelcast.core.HazelcastInstance;
import com.hazelcast.map.IMap;
import java.util.List;
import java.util.Properties;
public class HazelcastClientDemo {
public static void main(String[] args) {
ClientConfig config = new ClientConfig();
// Start the Hazelcast Client and connect to an already running Hazelcast Cluster on 127.0.0.1
//HazelcastInstance hz = HazelcastClient.newHazelcastClient();
ClientNetworkConfig clientNetworkConfig = config.getNetworkConfig();
Properties properties = new Properties();
properties.put("hazelcast.instance", "dev");
properties.put("hazelcast.clustername", "hz-test");
properties.put("hazelcast.password", "");
clientNetworkConfig.setSSLConfig(new SSLConfig()
.setEnabled(false)
.setProperties(properties));
clientNetworkConfig.setAddresses(List.of("localhost:5701"));
config.setClusterName("dev");
config.setInstanceName("hz-test");
HazelcastInstance hazelcastInstance = HazelcastClient.newHazelcastClient(config);
IMap<String, String> testMap = hazelcastInstance.getMap("test-map");
testMap.addEntryListener(new DemoEntryListener(), true);
System.out.println("Size before add : "+testMap.size());
testMap.put("1", "JavaTechARC3i");
System.out.println("Size add add : "+testMap.size());
System.out.println("Map Data : "+testMap);
IQueue<String> iQueue = hazelcastInstance.getQueue("employee_queue");
iQueue.add("Test");
iQueue.remove("Test");
}
}
Alternatively, you can configure listeners via XML files, which is useful for larger applications where centralized configuration is preferred. XML configuration allows developers to manage listeners without embedding logic directly into the code.
Use Cases for Hazelcast Listeners
Hazelcast listeners can be applied in various scenarios, improving performance and reliability in distributed systems:
- Real-time Data Synchronization:
Listeners ensure that when data is updated in one node, all other nodes in the cluster receive the same update instantly, ensuring data consistency. - Logging and Monitoring:
You can use listeners to log important events, such as when entries are added to or removed from a map. This is helpful for tracking application behavior and debugging. - Triggering Automated Actions:
Listeners can be configured to trigger specific actions when certain events occur. For instance, aMessageListener
can send notifications when a new message is published to a topic.
These use cases highlight the versatility and importance of listeners in ensuring efficient event handling and data management across a Hazelcast cluster.
Benefits of Using Hazelcast Listeners
- Real-Time Monitoring Hazelcast listeners provide real-time insights into data changes, enabling more dynamic and responsive applications.
- Event-Driven Architecture By leveraging listeners, you can implement an event-driven architecture that allows applications to react automatically to specific data events.
- Reduced Latency Since listeners are part of the in-memory architecture of Hazelcast, they help reduce latency by instantly responding to events without external database calls.
- Simplified Application Logic Using listeners simplifies your application logic by moving data-change monitoring to Hazelcast, reducing the need for polling or manual checks.
Best Practices for Using Hazelcast Listeners
To ensure optimal performance and reliability when using listeners in Hazelcast, consider the following best practices:
- Avoid Heavy Business Logic in Listeners:
Listeners should be lightweight to avoid performance bottlenecks. Heavy business logic can slow down event processing, impacting the entire system’s responsiveness. - Leverage Asynchronous Listeners:
For systems that require high throughput, consider using asynchronous listeners. These listeners process events in a non-blocking manner, allowing the main application flow to continue uninterrupted. - Implement Error Handling:
Distributed systems are prone to network failures or node crashes. Ensure that your listeners have proper error handling to deal with potential issues that might arise during event propagation.
Following these practices will help maintain a smooth and responsive distributed system, with listeners playing a key role in managing real-time events.
Conclusion
Hazelcast listeners are essential for handling distributed events in real-time. They help synchronize data, trigger automated actions, and maintain system responsiveness across clusters. By configuring listeners correctly and following best practices, developers can build distributed systems that are both efficient and resilient.
Hazelcast listeners offer a flexible and powerful way to monitor and respond to changes within a distributed system, making them an invaluable tool for any developer working with distributed data management.
The Hazelcast Listeners events sample code available on github.