Overview
gRPC ManagedChannel with SSL/TLS is the core communication channel between a gRPC client and a gRPC server. It manages the connection, load balancing, retries, and handles the lifecycle of network connections. When working with secure communications, it’s important to configure this channel with SSL/TLS for encryption and authentication.
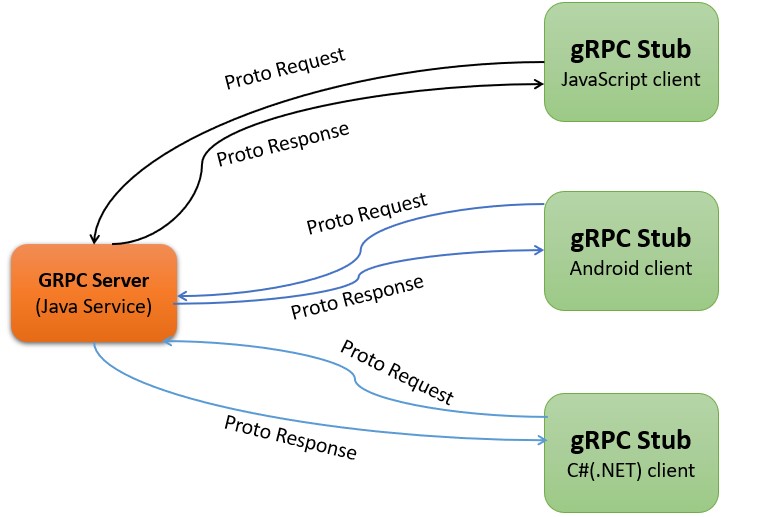
In this guide, we will explore how ManagedChannel
works and how to configure SSL/TLS for secure communication.
Know more about basic:
What is gRPC ManagedChannel
?
A gRPC ManagedChannel
is a client-side communication endpoint used to send requests to a gRPC server. It abstracts the complexities of network communication and provides essential features like:
- Connection Management: It handles the creation and reuse of network connections between the client and server.
- Load Balancing: If there are multiple server instances,
ManagedChannel
can distribute client requests among them. - Name Resolution: Dynamically resolves server addresses from a name (e.g., DNS).
- Retries: Automatically handles retries in case of connection failures.
- Security: Supports encrypted communication using SSL/TLS.
Creating a ManagedChannel
Here’s how you typically create a simple ManagedChannel
in Java without security:
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 8090)
.usePlaintext() // No SSL (not secure)
.build();
What is SSL/TLS in gRPC?
SSL (Secure Sockets Layer) and TLS (Transport Layer Security) are cryptographic protocols that provide encrypted communication over a network. When gRPC ManagedChannel with SSL/TLS uses it ensures that:
- Data exchanged between the client and server is encrypted and cannot be intercepted or altered.
- The server’s identity is verified using certificates, preventing man-in-the-middle attacks.
- (Optional) Mutual TLS can be used to authenticate both the client and the server.
In a typical gRPC setup, SSL/TLS requires the server to present an SSL certificate to the client, and the client verifies this certificate to establish trust.
How gRPC ManagedChannel with SSL/TLS Works
- TLS (Transport Layer Security) provides encryption for data in transit.
- The server holds an SSL certificate to authenticate its identity to clients.
- The client verifies the server’s certificate, establishing trust.
- Optionally, mutual TLS (mTLS) can be used, where both the server and client authenticate each other using certificates.
Types of gRPC ManagedChannel with SSL/TLS Configurations
- One-way SSL/TLS: Only the server presents a certificate to the client for authentication.
- Mutual TLS (mTLS): Both the client and server present certificates to each other for mutual authentication.
How to Configure gRPC ManagedChannel with SSL/TLS
To enable SSL/TLS in gRPC, you need to configure the ManagedChannel
with a trusted certificate. Below are the steps for configuring SSL/TLS.
Step 1: Obtain the SSL Certificate
The server needs an SSL certificate (usually a .crt
file) to authenticate itself to clients. This certificate can either be:
- A self-signed certificate (for testing and development)
- A certificate signed by a trusted Certificate Authority (CA) (for production)
Step 2: Install gRPC and Dependencies
Make sure you have the necessary gRPC libraries installed in your project:
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty-shaded</artifactId>
<version>1.66.0</version>
</dependency>
The grpc-netty-shaded
library allows you to configure gRPC ManagedChannel
with SSL/TLS.
Step 3: Configuring SSL/TLS on gRPC Server
In this example, we will configure a secure ManagedChannel
with SSL/TLS, where the client verifies the server’s identity.
package com.javatecharc.demo.grpc;
import com.javatecharc.demo.grpc.service.HelloWorldService;
import io.grpc.Server;
import io.grpc.ServerBuilder;
import java.io.File;
import java.io.IOException;
public class HelloWorldServerSSL {
private final Server server;
public HelloWorldServerSSL(int port) throws IOException {
//TODO : SSL Configuration on gRPC Server side
server = ServerBuilder.forPort(8443)
.useTransportSecurity(new File("server.crt"), new File("server.pem"))
.addService(new HelloWorldService())
.build();
server.start();
}
public void start() throws IOException {
server.start();
System.out.println("GRPC Server started on port " + server.getPort());
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
System.err.println("*** Shutting down gRPC server since JVM is shutting down");
HelloWorldServerSSL.this.stop();
System.err.println("*** Server shut down");
}));
}
public void stop() {
if (server != null) {
server.shutdown();
}
}
public void blockUntilShutdown() throws InterruptedException {
if (server != null) {
server.awaitTermination();
}
}
public static void main(String[] args) throws IOException, InterruptedException {
HelloWorldServerSSL server = new HelloWorldServerSSL(8090);
server.start();
server.blockUntilShutdown();
}
}
Explanation:
trustManager(serverCert)
: This configures the client to trust the server’s SSL certificate. The client will verify the certificate to ensure it’s connecting to the right server.sslContext(sslContext)
: Configures the channel to use SSL/TLS for secure communication.shutdown()
: Always ensure that you clean up and close the channel when you’re done.
Step 4: Configuring SSL/TLS on gRPC client
Once your server is set up, the next step is to configure the client to use SSL/TLS to communicate securely with the server.
package com.javatecharc.demo.grpc.client;
import com.javatecharc.demo.proto.HelloRequest;
import com.javatecharc.demo.proto.HelloResponse;
import com.javatecharc.demo.proto.HelloWorldGrpc;
import io.grpc.ManagedChannel;
import io.grpc.netty.shaded.io.grpc.netty.GrpcSslContexts;
import io.grpc.netty.shaded.io.grpc.netty.NettyChannelBuilder;
import io.grpc.netty.shaded.io.netty.handler.ssl.SslContext;
import javax.net.ssl.SSLException;
import java.io.File;
public class HelloWorldClientSSL {
public static void main(String[] args) throws SSLException {
// Path to the server's trusted certificate
File serverCert = new File("path/to/server.crt");
// Create SSL context using the server's certificate
SslContext sslContext = GrpcSslContexts.forClient()
.trustManager(serverCert)
.build();
ManagedChannel managedChannel = NettyChannelBuilder.forAddress("localhost", 8443)
.sslContext(sslContext)
.maxInboundMessageSize(10 * 1024 * 1024)
.build();
HelloWorldGrpc.HelloWorldBlockingStub stub = HelloWorldGrpc.newBlockingStub(managedChannel);
HelloResponse response = stub.helloJavaTechArc(HelloRequest.newBuilder().setName("Java TechARC 3i").build());
System.out.println("Response from server: " + response.getMessage());
managedChannel.shutdown();
}
}
Explanation:
trustManager(serverCert)
: Configures the client to trust the server’s certificate.maxInboundMessageSize(int)
: Maximum size of message flow via channel- Both client and server verify each other’s identity, ensuring a more secure communication channel.
Best Practices for Using SSL/TLS in gRPC
- Use Certificates Signed by a Trusted Certificate Authority (CA):
- For production, avoid self-signed certificates and use certificates signed by trusted CAs.
- Verify the Server’s Identity:
- Always ensure the client properly verifies the server’s certificate to prevent man-in-the-middle attacks.
- Renew Certificates Before Expiry:
- Keep track of certificate expiration dates and ensure they are renewed in advance.
- Test Certificate Configuration:
- Before deploying to production, thoroughly test SSL/TLS configurations, including both one-way SSL and mutual TLS.
- Use Mutual TLS for Enhanced Security:
- If both client and server need to authenticate each other, use mutual TLS to provide an additional layer of security.
Conclusion
ManagedChannel
is the foundation of gRPC client-server communication, and configuring it gRPC ManagedChannel with SSL/TLS ensures that the communication remains secure. Whether you use one-way SSL/TLS for basic encryption or mutual TLS (mTLS) for enhanced security, SSL/TLS configuration is crucial for protecting sensitive data and preventing unauthorized access. By following the steps outlined in this guide, you can confidently secure your gRPC channels in production environments.
gRPC server ManagedChannel with SSL/TLS sample code available on Github.