Overview
gRPC has rapidly gained popularity in the world of microservices due to its high performance, efficiency, and cross-language support. By using Protocol Buffers (protobuf) for data serialization and HTTP/2 for communication, gRPC offers a modern, efficient, and scalable way to build distributed systems.
In this blog, we’ll walk through building your first gRPC Hello World Example application in Java. We will cover everything from setting up the environment to writing the service definition and implementing both the server and client.
Why gRPC?
Before we dive into the code, let’s briefly talk about why you should consider using gRPC for your applications. gRPC excels in microservices architecture because it offers:
- Low-latency communication: Based on HTTP/2, which enables multiplexing and reduces overhead.
- Efficient data serialization: Protocol Buffers provide smaller payloads and faster serialization compared to text-based formats like JSON.
- Cross-language support: You can create services in one language and consume them in another with ease.
- Streaming support: Allows for real-time data transmission via server-side, client-side, or bidirectional streaming.
Pre-Rrequisite
- Basics about protobuf
- Protocol Buffer Maven Plugins
- Basic understanding about protobuf data types
- Knowledge about Java and Maven
Now that we know why gRPC is a great choice, let’s get started on building your first application.
Setting Up the Environment
Step 1: Creating a Maven Project
In gRPC Hello World Example first, create a new Maven project by using IDE (Intellj, Eclipse, etc) or you can create it by Maven commands, If you don’t have Maven installed, follow the official instructions. Once you have Maven, create a simple project structure by running the following command:
mvn archetype:generate -DgroupId=com.example -DartifactId=grpc-hello-world -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Maven Project Structure
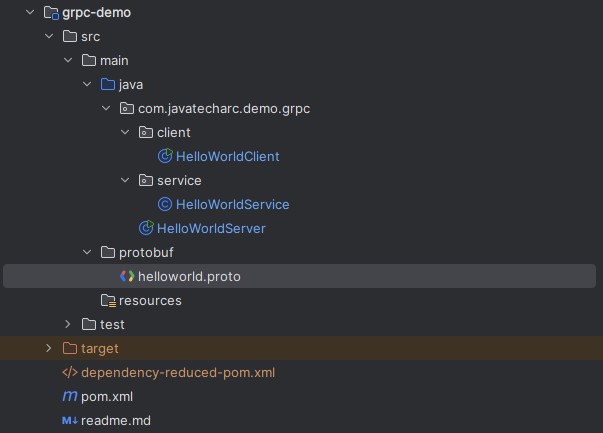
Step 2: Adding gRPC and Protobuf Dependencies
Next, In gRPC Hello World Example. we need to add the necessary dependencies for gRPC Hello World Example and Protocol Buffers in our pom.xml
. Add the following entries under the <dependencies>
section:
<dependencies>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty-shaded</artifactId>
<version>1.55.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>1.55.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>1.55.0</version>
</dependency>
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>3.19.0</version>
</dependency>
</dependencies>
We also need a protobuf Maven plugin to generate the necessary Java code from our Protocol Buffers definitions. Add the following under the <build>
section:
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<extensions>true</extensions>
<version>0.6.1</version>
<configuration>
<protoSourceRoot>src/main/protobuf</protoSourceRoot>
<protocArtifact>com.google.protobuf:protoc:3.21.6:exe:${os.detected.classifier}</protocArtifact>
<pluginId>grpc-java</pluginId>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:1.53.0:exe:${os.detected.classifier}</pluginArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
Defining the gRPC Service with Protocol Buffers
In gRPC Hello World Example, services are defined using Protocol Buffers (proto files), which describe the structure of the messages and the RPC methods. Let’s create a simple Hello World service.
Step 3: Writing the Service Definition
Create a new file named helloworld.proto
under the src/main/proto
directory. Add the following content:
syntax = "proto3";
option java_multiple_files = true;
option java_package = "com.javatecharc.demo.proto";
option java_outer_classname = "HelloWorldProto";
option optimize_for = SPEED;
service HelloWorld {
rpc helloJavaTechArc (HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
This defines a HelloWorld
service with a single method helloJavaTechArc
, which takes a HelloRequest
and returns a HelloResponse
.
Step 4: Generating the Java Code
To generate the necessary Java classes from our hello.proto
file, run the following Maven command:
mvn clean install
This will create the Java classes under the target that will act as stubs for our client and service implementation like below
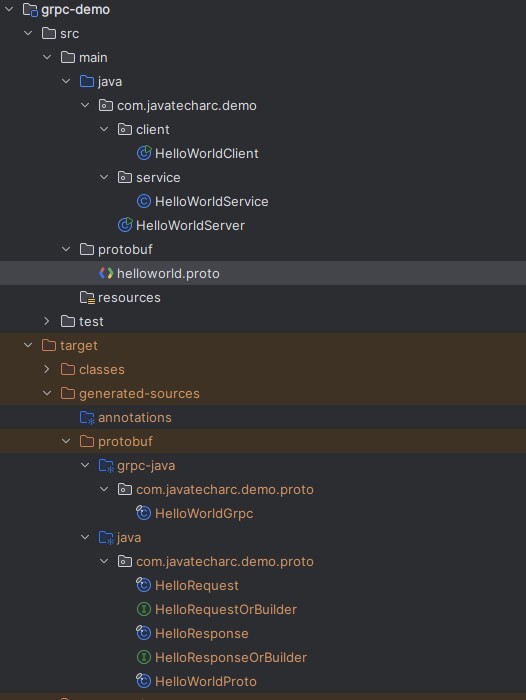
Implementing the gRPC Server
Now, we’ll implement the server-side logic of the Hello World application.
Step 5: Creating the Server
Create a new class HelloWorldServer
under the com.javatecharc.demo.grpc package:
package com.javatecharc.demo.grpc;
import com.javatecharc.demo.grpc.service.HelloWorldService;
import io.grpc.Server;
import io.grpc.ServerBuilder;
import java.io.IOException;
public class HelloWorldServer {
private final Server server;
public HelloWorldServer(int port) {
server = ServerBuilder.forPort(port)
.addService(new HelloWorldService())
.build();
}
public void start() throws IOException {
server.start();
System.out.println("GRPC Server started on port " + server.getPort());
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
System.err.println("*** Shutting down gRPC server since JVM is shutting down");
HelloWorldServer.this.stop();
System.err.println("*** Server shut down");
}));
}
public void stop() {
if (server != null) {
server.shutdown();
}
}
public void blockUntilShutdown() throws InterruptedException {
if (server != null) {
server.awaitTermination();
}
}
public static void main(String[] args) throws IOException, InterruptedException {
HelloWorldServer server = new HelloWorldServer(8090);
server.start();
server.blockUntilShutdown();
}
}
This server listens on port 8090 and responds with a “Hello” message to any client request.
Step 6: Creating a HelloWorldService
HelloWorldService
will have implementation of In gRPC Hello World Example endpoint method helloJavaTechArc
, which will be the implementation of generate classes HelloWorldGrpc.HelloWorldImplBase
package com.javatecharc.demo.grpc.service;
import com.javatecharc.demo.proto.HelloRequest;
import com.javatecharc.demo.proto.HelloResponse;
import com.javatecharc.demo.proto.HelloWorldGrpc;
import io.grpc.stub.StreamObserver;
public class HelloWorldService extends HelloWorldGrpc.HelloWorldImplBase {
@Override
public void helloJavaTechArc(HelloRequest req, StreamObserver<HelloResponse> responseObserver) {
HelloResponse response = HelloResponse.newBuilder().setMessage("Welcome to '"+req.getName()+"' GRPC Hello World Demo!" ).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
Implementing the gRPC Client
To test our server, we’ll implement a simple client.
Step 7: Creating the Client
In In gRPC Hello World Example, Create a new class HelloWorldClient
in the package:
package com.javatecharc.demo.grpc.client;
import com.javatecharc.demo.proto.HelloRequest;
import com.javatecharc.demo.proto.HelloResponse;
import com.javatecharc.demo.proto.HelloWorldGrpc;
import io.grpc.ManagedChannel;
import io.grpc.ManagedChannelBuilder;
public class HelloWorldClient {
public static void main(String[] args) {
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 8090)
.usePlaintext()
.build();
HelloWorldGrpc.HelloWorldBlockingStub stub = HelloWorldGrpc.newBlockingStub(channel);
HelloResponse response = stub.helloJavaTechArc(HelloRequest.newBuilder().setName("Java TechARC 3i").build());
System.out.println("Response from server: " + response.getMessage());
channel.shutdown();
}
}
Step 8: Running the Server
In one terminal, run the following command to start the server, also you use the IDE to start the main server class:
mvn exec:java -Dexec.mainClass="com.javatecharc.demo.grpc.HelloWorldServer"
Step 9: Running the Client
In a separate terminal, run the client with the following command, also you use the IDE to start the main server class:
mvn exec:java -Dexec.mainClass="com.javatecharc.demo.grpc.client.HelloWorldClient"
If everything is set up correctly, the client will connect to the server, send a “World” request, and print the server’s response:
Response from server: Welcome to 'Java TechARC 3i' GRPC Hello World Demo!
Conclusion
In this blog, we’ve walked through building a gRPC Hello World example in Java, starting from setting up the environment, defining the service using Protocol Buffers, to implementing both the server and client. This simple example serves as a foundation for understanding how gRPC works in Java. You can now expand this to more complex use cases, such as bidirectional streaming or adding authentication to your gRPC services.
gRPC Hello World sample code available on Github.